PWA stands for Progressive Web Apps: the very progressive combination of native and the web. They provide a quick response, work offline, send push notifications and can be installed on the device like normal applications. In this article by Celadonsoft, we will cover the React PWA Tutorial, following the step-by-step guide.
Why Use PWA?
PWAs, according to the Celadonsoft’s experience in https://celadonsoft.com/mvp-development MVP development services, offer a number of benefits for both developers and users:
- Cross-platform – one application for mobile and desktop devices.
- Performance – thanks to caching and optimization of content loading.
- Offline access – the application works even when no internet is available.
- Cost-effective – faster and cheaper than native application.
Web Application Manifest Settings
The main features of the React PWA Tutorial include something called Web App Manifest-a manifest.json file, which determines how an application should behave once it’s installed on a user’s device. It gives you a chance to define the name of your application, icons, color scheme, display mode, and many other settings that will make the user’s experience with the app even more polished.
1. Creating manifest.json
When you create a new project by using Create React App, this manifest is in the standard configuration and can be found in /public/manifest.json. At the same, it needs customization for the specific application.
2. Analysis of Key Parameters
- name and short_name are the full and short names of the application displayed during installation.
- icons are icons for various devices and screen resolutions.
- start_url – URL from which the application will be run after installation.
- display – application display mode:
- standalone – as a separate application without browser elements;
- fullscreen – full screen
- minimal-ui – with a minimal set of browser UI elements;
- browser – The normal mode of the browser.
- theme_color is the main color used in the OS browser and UX.
- background_color is the background color when the application is loaded.
3. Connecting a Manifest to React
To have the browser recognize manifest.json, you need to connect it to public/index.html.
In the React application created with Create React App, this tag is already added by default.
4. Adding an Application Icon
For proper PWA installation on devices and user convenience, it is important to prepare several versions of icons in different resolutions.
Recommended sizes:
- 192×192 – for Android devices;
- 512×512 – to install on the main screen and in the application list.
The icons are placed in the /public/icons/ folder and specified in manifest.json.
5. Checking the Correctness of the Manifest
After configuration, it is influential to make sure that the manifest is correctly recognized. For this:
- Open Chrome DevTools (F12 -> tab Application).
- In the Manifest section, check if all settings and icons are loaded.
- Make sure the application supports installation – in DevTools you should see the section “Installability”.

Registering a Service Worker in React PWA
One of the key components of React PWA Tutorial
is the Service Worker – a background script that allows you to cache resources, provide offline access and manage network requests. Let’s now describe how to configure and register Service Worker in React applications.
Service Worker: what is it and why do I need it?
Service Worker is a JavaScript file that runs separately from the so-called main thread, which is performing execution. It can:
- Intercept and manage HTTP requests,
- cache static and dynamic resources,
- send push notifications,
- work even when no internet is available.
The PWA Service Worker is key to ensuring high performance and stability of the application.
Service Worker in the Create React App
If the application is created using Create React App (CRA), then the basic Service Worker configuration is already enabled. By default, CRA uses the Workbox library to register a Service Worker, but it is disabled in development mode and only activated in production.
The project structure already contains a file src/service-worker.js which describes the basic working methods.
Registering a Service Worker in React
To enable the Service Worker, open src/index.js and replace the string:
serviceWorker.unregister();
on:
serviceWorker.register();
The service worker will be registered when you start the application in the extension.
Service Worker Customization
Although CRA provides the basic configuration, in a real project the service worker is often configured manually. For example, you can add custom caching strategies:
self.addEventListener('fetch', (event) => {
event.respondWith(
caches.match(event.request). then(response) => {
return response || fetch(event.request);
})
);
});
This code checks if the requested resource is in cache. If yes, it is downloaded locally, if not, a network request is made.
Service Worker Performance Check
To make sure that the Service Worker is working, open DevTools in your browser (F12) -> under the Application tab -> Service Workers section. Here you can:
- check the Service Worker status,
- update or remove it,
- test offline behavior.
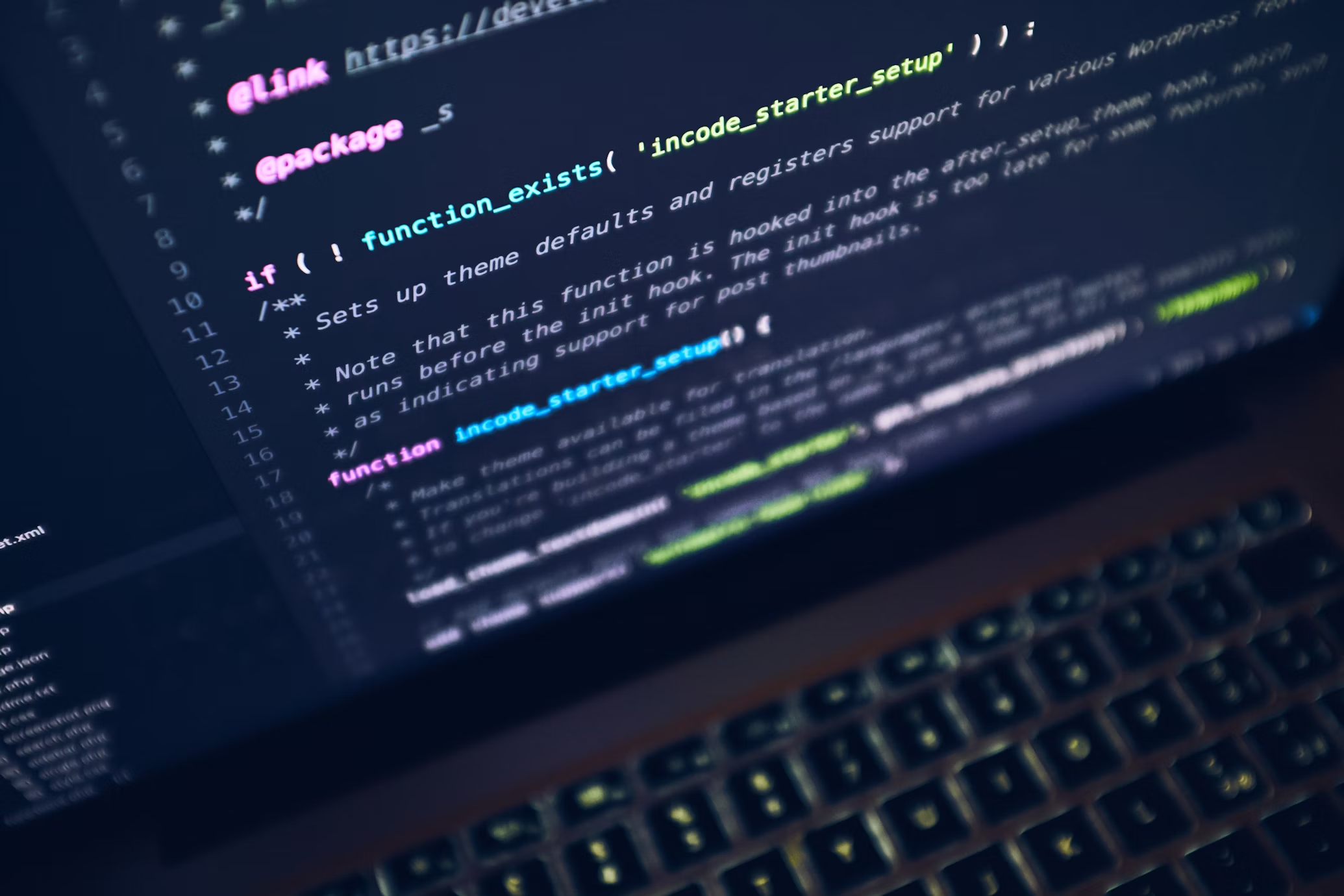
Offline Support
First and foremost, PWA allows it to work even without the network. Therefore, in the case of bad or unstable Internet, this application is more reliable for users. In React PWA, the offline functionality is provided by the Service Worker and the cache mechanism.
Caching Static Resources
To make the application load even when no network is available, it is necessary to cache its main resources, such as:
- HTML, CSS and JS files,
- images,
- fonts,
- API requests (if necessary).
In React PWA, static file caching can be implemented with the Workbox library, which simplifies working with the Service Worker. When creating a project with Create React App, the Workbox is already integrated and automatically configures caching.
If you require custom logic, you can change the settings to src/service-worker.js or override the work of the Workbox using workbox-routing and workbox-strategies.
Working With Dynamic Data Offline
Caching static resources is only half the task. It is also important to think about how the interaction with the server will work when there is no connection.
1. API request caching strategies
- Network First – first sends the request to the network if the server is unavailable – uses cache. Suitable for rarely updated data.
- Cache First – first uses the cache, if no data – requests them from the server. Useful for immutable data.
- Stale While Revalidate – uses cached data immediately, but in parallel makes a request to the server for cache update. Optimal for content to be updated, but not critical to get a fresh version right away.
2. Using IndexedDB for data storage
For complex offline scenarios, such as when the user needs to interact with dynamic content (create records, edit data), you can use IndexedDB. This allows to save the data locally, and when reconnecting – send them to the server. This approach allows users to continue working with the application even without the internet.
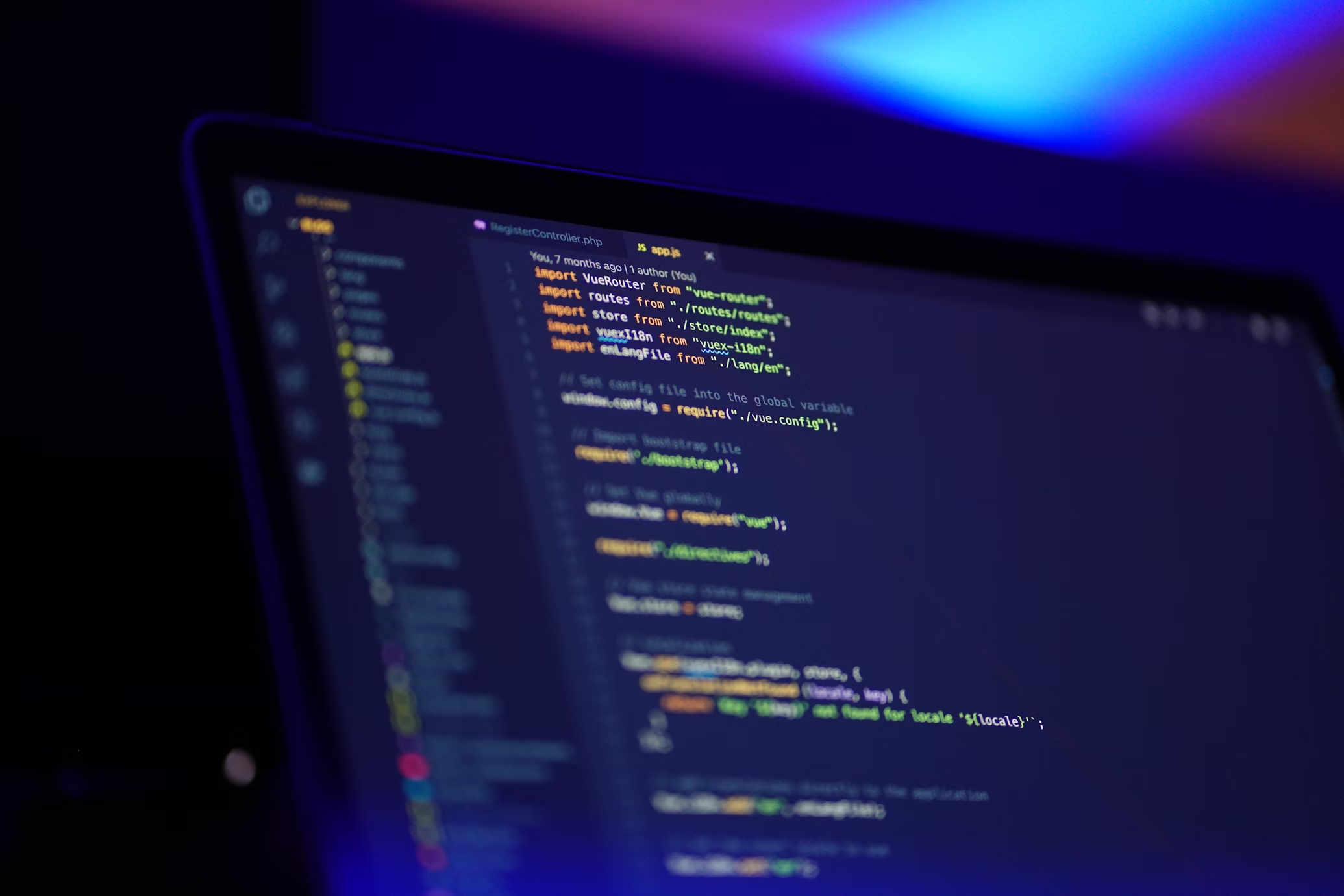
Add to Main Screen and Display Settings
For your PWA to look and function like a native application, you need to properly configure the display settings and allow the user to add it to the main screen.
The manifest.json file is a key PWA element that determines how an application will look when installed on a device. You can set:
- Application name (name) – the full name displayed during installation.
- Short name (short_name) – used for desktop icons.
- Theme color (theme_color) – defines the color of the browser panel.
- Background color (background_color) – the background of the boot screen.
- Display mode (display) – determines how the application is opened:
- standalone – works as native application without address line.
- fullscreen – hides all system elements of the interface.
- minimal-ui – Displays the minimum set of browser elements.
- Start URL (start_url) – specifies the page that is loaded at startup.
- Icons (icons) – images for different screens and sizes.
Improve Performance and Security
The Progressive Web Application (PWA) should not only work offline, but also provide fast uploading and security of user data. Consider key aspects of optimization.
Optimization of Performance
- Caching and loading resources: Use the Workbox for flexible static and dynamic data caching. This will reduce the loading time of pages and increase the responsive ability of the application.
- Code splitting and lazy downloads: Break the code into small chunks using React.lazy() and React Suspense to load components only when needed.
- Image optimization: Use modern formats (WebP, AVIF), compression and adaptive image upload to reduce network load.
Security
- Using HTTPS: PWAs should work through a secure connection. This not only protects the data, but is also a mandatory requirement for installing PWA on users’ devices.
- Service Worker Update
- Regularly update the Service Worker to remove outdated code versions and improve security.
- Protection against XSS and CSRF: Use security headers, Content Security Policy (CSP) and protection mechanisms against cross-site attacks.
PWA Testing and Debugging
Before releasing the application, it is important to perform a comprehensive test of the application, checking that it meets the PWA requirements.
Using Lighthouse for Auditing
Google Lighthouse is a tool to evaluate performance, accessibility, SEO, and compliance with PWA standards. You can run the analysis directly in Chrome DevTools.
Key figures:
- Boot speed
- Offline availability
- Mobile device support
- Compliance with PWA recommendations
Developer Tools
- DevTools (Application Service Workers) – check the state of the Service Worker and caching.
- Workbox Debugging – Service Worker loging.
- PWA Builder – helps you to quickly test and generate manifest files and Service Workers.
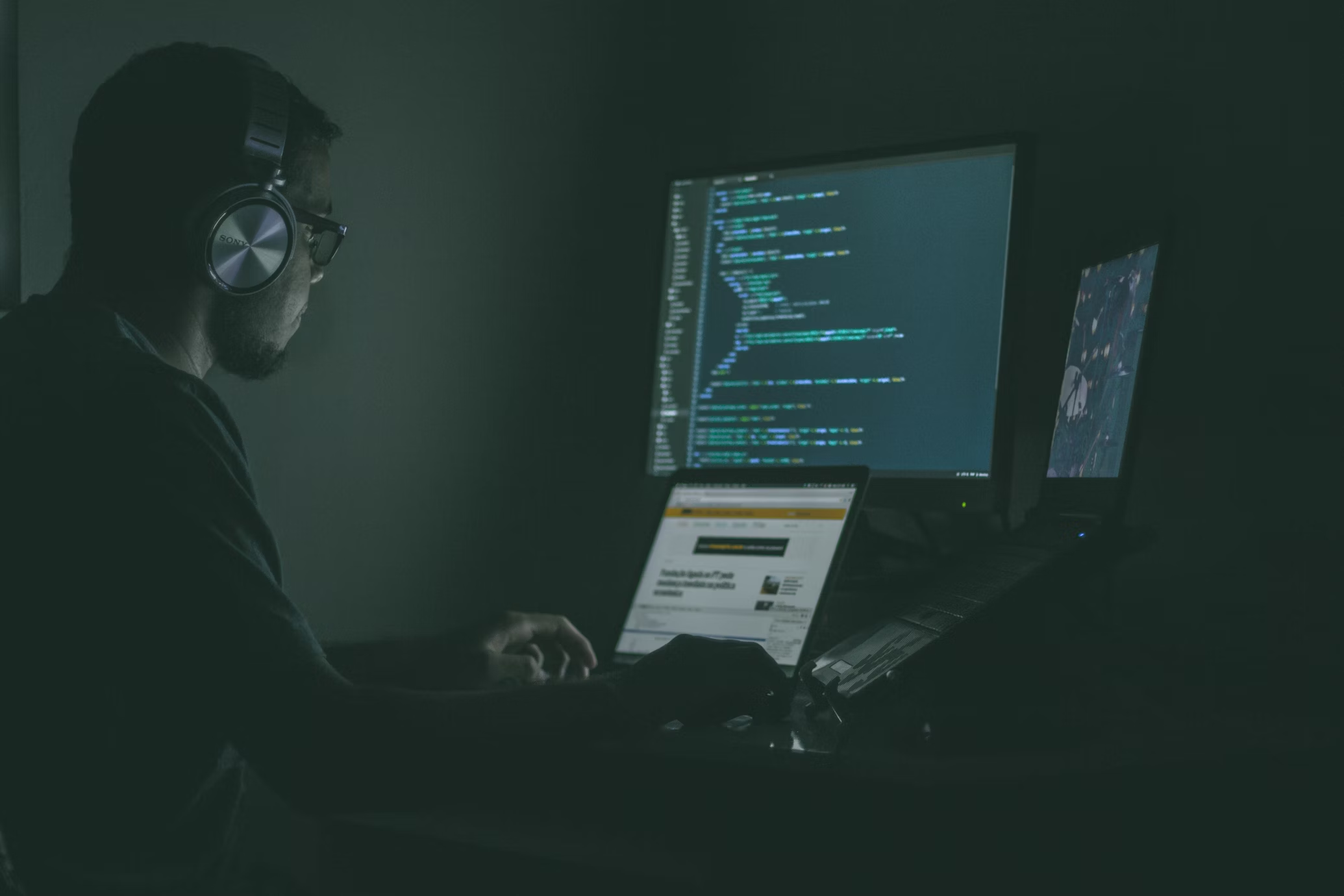
Default and Support App
Once the PWA on React is finished, it is important to take care of its proper deployment and further support. This section will cover the key steps of the application’s deployment and monitoring.
Choosing a Hosting
You must use HTTPS hosting to host the PWA (as the service worker only works in a secure environment). Consider popular solutions:
- Vercel is a convenient service with an automatic lock from the Git repository.
- Netlify – supports automatic deployment and provides built-in HTTPS.
- Firebase Hosting – from Google, great for PWA and supports serverless functions.
- GitHub Pages is a good option for static applications, but requires additional configuration for PWA.
Preparation for the Deployment
There are several steps to follow before publishing the application:
1. Optimization of assembly
- Make sure the application is assembled in production mode:
npm run build
- Use tools like Lighthouse to analyze performance.
2. Service worker update
- Set up the correct caching and upgrade strategy.
- Ensure that users receive the latest version of the application without having to restart.
3. Configuration manifest.json
Check the correctness of the manifesto, make sure that the application can be installed as PWA.
How to Host an Application
For default to the chosen hosting, follow its documentation. For example, for Vercel the process is simple:
npm install -g vercel
vercel
For Firebase Hosting:
npm install -g firebase-tools
firebase login
firebase init
firebase deploy
Monitoring and Updating
After deployment, it is important to monitor the stability and performance of the application:
- Logics and analytics
- Use Google Analytics or LogRocket to analyze user behavior.
- Connect Sentry to track bugs.
- Automatic update
- Implement mechanisms to notify users about new version.
- Configure the caching strategy to avoid problems with outdated versions.
- Regular updates
- Keep track of dependencies and update them as needed.
- Periodically test the application on different devices and in different browsers.